Mastering Python Exception Handling: Unveiling 5 Surprising Tips
Written on
Chapter 1: Introduction to Exception Handling
Did you realize that it's possible to capture multiple exceptions in just one line? Understanding Python's exception handling can greatly enhance your coding skills.
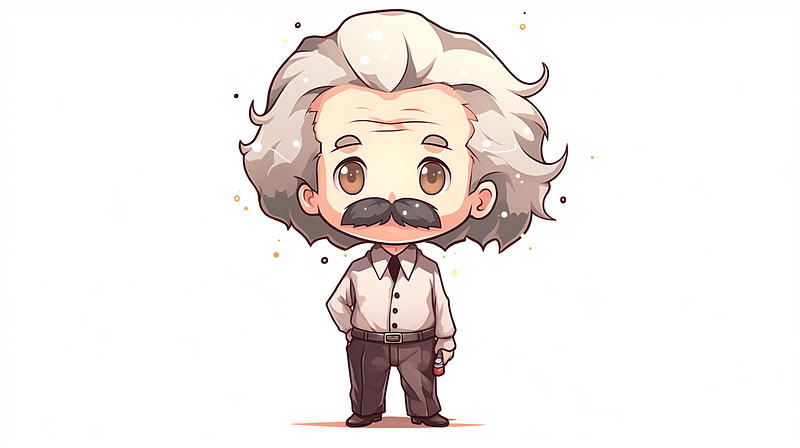
Custom Exceptions for Enhanced Clarity
Rather than exclusively depending on Python’s built-in exceptions, consider developing custom ones tailored to specific situations within your application. This approach improves code readability and provides clearer error messages.
class NegativeNumberError(Exception):
def __str__(self):
return "Negative numbers are not permitted!"
def check_positive(number):
if number < 0:
raise NegativeNumberError
The Utility of the else Clause in Exception Handling
A lot of people, myself included prior to writing this article, are not aware of the else clause in exception handling. This clause executes only when the try block does not throw any exceptions, leading to cleaner code.
try:
result = 10 / 2
except ZeroDivisionError:
print("Division by zero is not allowed!")
else:
print(f"The result is {result}")
Utilizing Logging Instead of Print for Errors
Rather than using print statements to report exceptions, leverage the logging module. This approach is more flexible and allows you to direct error messages to files or other outputs.
import logging
logging.basicConfig(level=logging.ERROR)
try:
# some code
except Exception as e:
logging.error(f"An error occurred: {e}")
Catching Multiple Exceptions in a Single Line
When facing multiple exceptions that require similar handling, you can catch them in one line, simplifying your code structure.
try:
# some code
except (ValueError, TypeError, IndexError) as e:
print(f"An error occurred: {e}")
Employing finally for Cleanup Operations
The finally block executes no matter whether an exception was raised, making it ideal for cleanup actions, such as closing files or releasing resources.
try:
file = open("example.txt", "r")
data = file.read()
except FileNotFoundError:
print("The specified file was not found!")
finally:
file.close()
Deepening Your Knowledge
For a comprehensive understanding of Python's exception handling, including best practices and common mistakes, take a look at this informative article.
Engage with Us!
Did any of these tips catch you off guard? Do you have a unique technique for generators that you'd like to share? Please leave your comments below so we can build a community of Python enthusiasts!
If you found this article valuable, I’d appreciate your support through claps (you can give up to 50) or likes. I’d also love to hear about any specific concepts that resonated with you or topics you'd like covered in future articles.
To keep updated with my latest writings and connect with me professionally, consider following me on Medium and LinkedIn. I’m always excited to expand my network and learn from fellow professionals.
Thank you for reading! I look forward to your feedback and connecting soon.
Chapter 2: Further Learning Resources
For those looking to deepen their understanding of error handling in Python, here are some recommended resources:
The first video offers a comprehensive tutorial on using try/except blocks for effective error handling.
The second video discusses best practices for error handling in Python, including the use of try/except/else/finally structures.