Create an Engaging Password Generator App Using Vue 3 and JS
Written on
Chapter 1: Introduction to Vue 3
Vue 3 is the newest iteration of the user-friendly Vue JavaScript framework, which empowers developers to build robust front-end applications. In this guide, we will explore the creation of a password generator app utilizing Vue 3 and JavaScript.
Create Your Project
To get started, we can set up the Vue project using Vue CLI. First, install it by executing the following command:
npm install -g @vue/cli
Alternatively, if you prefer Yarn, you can run:
yarn global add @vue/cli
Next, initiate your project by executing:
vue create password-generator
Make sure to choose all the default options during the setup process.
Create the Password Generator
To develop the password generator application, we will write the following code:
<template>
<form @submit.prevent="generatePassword">
<div>
<label>Length</label>
<input v-model.number="length" />
</div>
<button type="submit">Generate Password</button>
</form>
<div>{{ password }}</div>
</template>
<script>
const string = "abcdefghijklmnopqrstuvwxyz";
const numeric = "0123456789";
const punctuation = "!@#$%^&*()_+~`|}{[]:;?><,./-=";
export default {
name: "App",
data() {
return {
length: 10,
password: "",
};
},
computed: {
formValid() {
const { length } = this;
return +length > 0;
},
},
methods: {
generatePassword() {
const { length, formValid } = this;
if (!formValid) {
return;}
let character = "";
let password = "";
while (password.length < length) {
const entity1 = Math.ceil(string.length * Math.random() * Math.random());
const entity2 = Math.ceil(numeric.length * Math.random() * Math.random());
const entity3 = Math.ceil(punctuation.length * Math.random() * Math.random());
let hold = string.charAt(entity1);
hold = password.length % 2 === 0 ? hold.toUpperCase() : hold;
character += hold;
character += numeric.charAt(entity2);
character += punctuation.charAt(entity3);
password = character;
}
password = password.split("").sort(() => 0.5 - Math.random()).join("");
this.password = password.substr(0, length);
},
},
};
</script>
In this code, we have a form that listens for the submit event through the @submit directive. The .prevent modifier allows us to perform client-side submission without refreshing the page. The form includes an input field linked to the length reactive property using v-model. The number modifier ensures that the bound value is treated as a number.
The submit button, which triggers the password generation, is located below the input field, and the generated password is displayed underneath the form.
Within the script section, we define string, numeric, and punctuation variables from which we derive characters to form the password. The data method returns the necessary reactive properties. The formValid property checks if the length exceeds zero to ensure that a valid password can be generated.
The generatePassword method first checks the validity of the form before proceeding to create the password. A while loop is employed to construct a password of the specified length by randomly selecting characters from the defined sets. The characters are then shuffled using the sort method to ensure randomness.
Finally, we assign the generated password, trimmed to the specified length, to the this.password reactive property.
Conclusion
Creating a password generator app with Vue 3 and JavaScript is straightforward and fun. If you enjoyed this guide, consider subscribing to our YouTube channel for more engaging content!
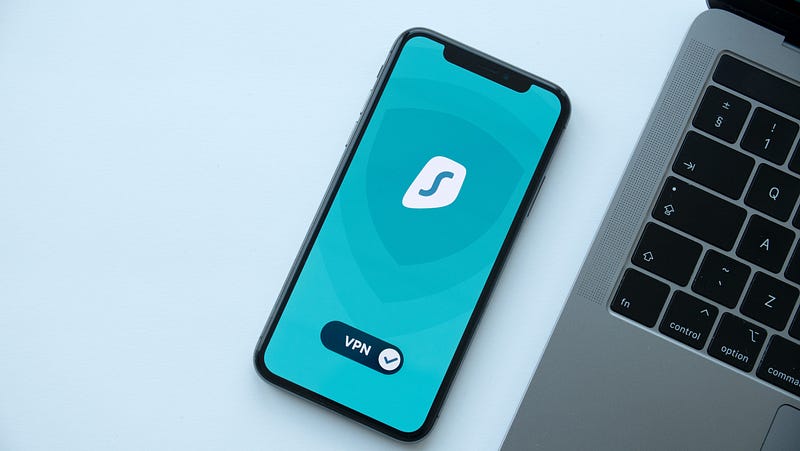
Photo by Dan Nelson on Unsplash
For further articles and resources, visit plainenglish.io.